NORMAL OR GAUSSIAN DISTRIBUTION
The random.normal() method is used to get a Normal Data Distribution.
It has three parameters-
loc – (Mean) where the peak of the bell exists.
scale – (Standard Deviation) how flat the graph distribution should be.
size – The shape of the returned array.
from numpy import random
x = random.normal(size=(2, 2))
print(x)
Output-
[[-1.15661419 -1.68760216]
[ 0.0067232 -0.20673282]]
Explanation–
random.normal(size=(2, 2)) – It will generate a random normal distribution of size 2×2
Example- To generate a random normal distribution of size 2×2 with mean at 1 and standard deviation of 2
from numpy import random
x = random.normal(loc=1, scale=2, size=(2, 2))
print(x)
Output-
[[-0.54095408 2.84654988]
[ 2.43039472 -1.06578449]]
Visualization of Normal Distribution
from numpy import random
import matplotlib.pyplot as plt
import seaborn as sns
sns.displot(random.normal(size=100))
plt.show()
Output-
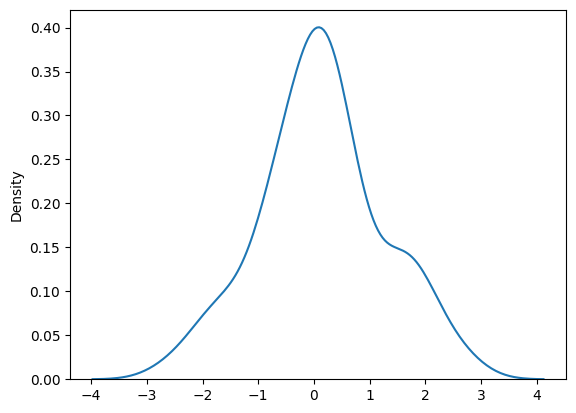
Leave a Reply