The uniform distribution is represented by the numpy.random.uniform function. It’s used to generate random numbers that are uniformly distributed over a specified interval. Used to describe probability where every event has equal chances of occuring. Here’s a breakdown of what it does:
Functionality:
Generates random floating-point numbers.
Numbers are distributed uniformly across a half-open interval [low, high). This means values are greater than or equal to low (inclusive) but strictly less than high (exclusive).
Parameters:
low (float, optional): The lower bound of the interval (inclusive). Defaults to 0.
high (float, optional): The upper bound of the interval (exclusive). Defaults to 1.0.
size (int or tuple of ints, optional): The desired output shape. If left as None (default), it returns a single value if low and high are scalars, otherwise an array with the same shape as low and high.
Example:
import numpy as np
#Generate 5 random numbers between 2 and 10 (exclusive)
random_samples = np.random.uniform(2, 10, 5)
print(random_samples)
#This code will print an array of 5 random floating-point numbers between 2 (inclusive) and 10 (exclusive).
Output
[3.82947451 6.47805218 7.67506037 6.57569189 9.81194928]
Key Points:
The uniform distribution ensures all values within the interval have an equal chance of being generated.
It’s useful for various simulations and experiments where you want random values within a specific range.
Additional Notes:
For discrete uniform distribution (generating random integers), you can use np.random.randint.
To get random samples from a uniform distribution over the interval [0, 1) (which is commonly used), you can directly use np.random.rand() or np.random.rand(size).
Visualisation of Uniform Distribution-
from numpy import random
import matplotlib.pyplot as plt
import seaborn as sns
sns.distplot(random.uniform(2,10,5), hist=False)
plt.show()
Output
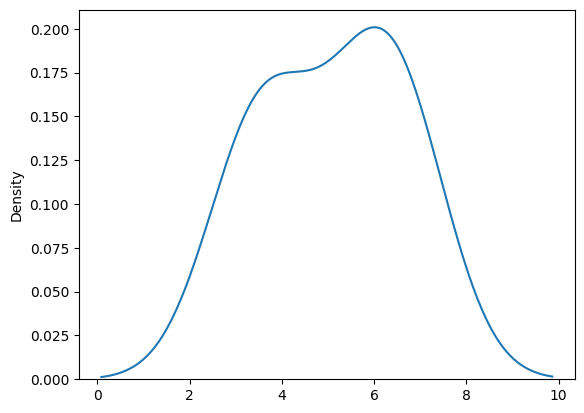
Leave a Reply