Poisson Distribution
The Poisson distribution is a discrete probability distribution that models the number of events occurring in a fixed interval of time or space, with a known average occurrence rate. It’s useful for situations where events happen independently and at a constant rate.
In Python’s NumPy library, you can generate random samples from a Poisson distribution using the np.random.poisson function. This function takes two arguments:
lam (float or array-like of floats): This is the average number of events expected in the interval. It must be non-negative.
size (int or tuple of ints, optional): This is the desired output shape. If left as None (default), it returns a single value if lam is a scalar, otherwise an array with the same size as lam.
Example
import numpy as np
# Example data
lambda_val = 5 # Average number of events
# Generate Poisson distributed random numbers with NumPy's random.poisson function
poisson_samples = np.random.poisson(lambda_val, 1000)
#1000 samples
# Print the first 10 samples
print(poisson_samples[:10])
Output
[ 6 7 2 4 5 7 4 10 8 5]
Visualization of Poisson Distribution
from numpy import random
import matplotlib.pyplot as plt
import seaborn as sns
sns.distplot(random.poisson(lam=5, size=1000), kde=False)
plt.show()
Output
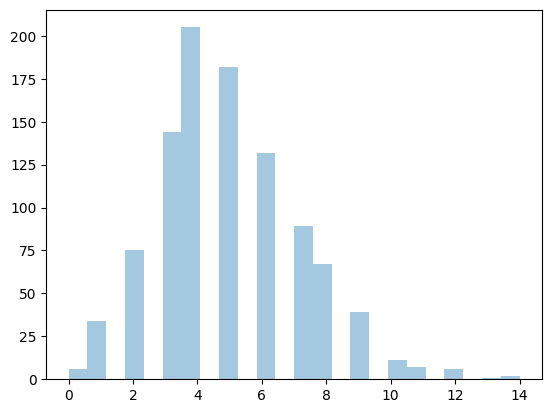
Leave a Reply