What are identifiers in java?
Identifiers are names in java program. Identifiers can be class name, method name or variable name. Rules for defining identifiers in java: 1) Identifiers must start with letter, Underscore or dollar($) sign.2) Identifiers canโt start with numbers .3) There is no limit on number of characters in identifier but not recommended to have more than […]
Can we define package statement after import statement in java?
We canโt define package statement after import statement in java. Package statement must be the first statement in source file. We can have comments before the package statement
Can we have more than one package statement in source file ?
We canโt have more than one package statement in source file. In any java program there can be atmost only 1 package statement. We will get compilation error if we have more than one package statement insource file.
What are packages in java?
Package is a mechanism to group related classes ,interfaces and enums in to a single module. Package can be declared using the following statement : Syntax : Coding Convention : package name should be declared in small letters. package statement defines the namespace. The main use of package is: 1) To resolve naming conflicts 2) […]
What all access modifiers are allowed for top class ?
For top level class only two access modifiers are allowed. public and default. If a class is declared aspublic it is visible everywhere.If a class is declared default it is visible only in same package.If we try to give private and protected as access modifier to class we get the below compilation error.Illegal Modifier for […]
Can we have multiple classes in single file ?
Yes we can have multiple classes in single file but it people rarely do that and not recommended. We canhave multiple classes in File but only one class can be made public. If we try to make two classes in Filepublic we get following compilation error.โThe public type must be defined in its own fileโ
What does null mean in java?
When a reference variable doesnโt point to any value it is assigned null.Example : Employee employee;In the above example employee object is not instantiate so it is pointed no where.
Explain about instanceof operator in java?
Instanceof operator is used to test the object is of which type. Syntax : instanceof Instanceof returns true if reference expression is subtype of destination type. Instanceof returns false if reference expression is null. Example : Since a is integer object it returns true.There will be a compile time check whether reference expression is subtype […]
Difference between โIS-Aโ and โHAS-Aโ relationship in java?
IS-A RELATIONSHIP HAS- A RELATIONSHIP Is a relationship also known as inheritance Has a relationship also known as composition or aggregation. For IS-A relationship we uses extends keyword For Has a relationship we use new keyword Ex : Car is a vehicle. Ex : Car has an engine. We cannot say Car is an engine […]
What is โHAS Aโโ relationship in java?
โHas a โ relationship is also known as โcomposition or Aggregationโ. As in inheritance we have โextendsโkeyword we donโt have any keyword to implement โHas aโ relationship in java. The main advantage ofโHas-Aโ relationship in java code reusability.
What is โIS-A โ relationship in java?
โis aโ relationship is also known as inheritance. We can implement โis aโ relationship or inheritance in javausing extends keyword. The advantage or inheritance or is a relationship is reusability of code instead ofduplicating the code.Ex : Motor cycle is a vehicleCar is a vehicle Both car and motorcycle extends vehicle.
Explain Java Coding Standards for Constants?
Constants in java are created using static and final keywords.1) Constants contains only uppercase letters.2) If constant name is combination of two words it should be separated by underscore.143) Constant names are usually nouns.Ex:MAX_VALUE, MIN_VALUE, MAX_PRIORITY, MIN_PRIORITY
Explain Java Coding Standards for variables ?
1) Variable names should start with small letters.2) Variable names should be nouns3) Short meaningful names are recommended.4) If there are multiple words every innerword should start with Uppecase character.Ex : string,value,empName,empSalary
Explain Java Coding standards for Methods?
1) Method names should start with small letters.2) Method names are usually verbs3) If method contains multiple words, every inner word should start with uppercase letter.Ex : toString()4) Method name must be combination of verb and nounEx : getCarName(),getCarNumber()
Explain Java Coding standards for interfaces?
1) Interface should start with uppercase letters2) Interfaces names should be adjectivesExample : Runnable, Serializable, Marker, Cloneable
Explain Java Coding Standards for classes or Java coding conventions for classes?
Sun has created Java Coding standards or Java Coding Conventions . It is recommended highly to followjava coding standards.Classnames should start with uppercase letter. Classnames names should be nouns. If Class name is ofmultiple words then the first letter of inner word must be capital letter.Ex : Employee, EmployeeDetails, ArrayList, TreeSet, HashSet
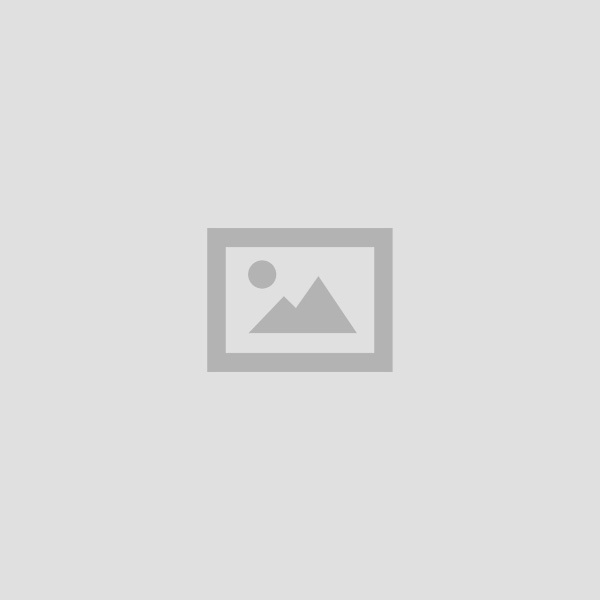
Difference between Right shift operator โ>>โ and Unsigned shift operator โ>>>โ in java?
The right shift operator ‘>>’ shifts all of the bits in a value to the right to a specified number of times.int a =15;a= a >> 3;The above line of code moves 15 three characters right. The unsigned shift operator ‘>>>’ used to shift right. The places which were vacated by shift are filledwith zeroes.
What are constants and how to create constants in java?
Constants are fixed values whose values cannot be changed during the execution of program. We createconstants in java using final keyword.Ex : final int number =10;final String str=โjava-interview โquestionsโ
What is difference between Character Constant and String Constant in java ?
Character constant is enclosed in single quotes. String constants are enclosed in double quotes. Characterconstants are single digit or character. String Constants are collection of characters.Ex :โ2โ, โAโEx : โHello Worldโ
What is Unicode ?
Unicode is a character set developed by Unicode Consortium. To support all languages in the world Javasupports Unicode values. Unicode characters were represented by 16 bits and its character range is 0-65,535.Java uses ASCII code for all input elements except for Strings, identifiers, and comments.